/* Program in C# to Input Two Double numbers from the user and find the Addition, Subtraction, Multiplication, Division, Remainder and factorial of the two numbers. */
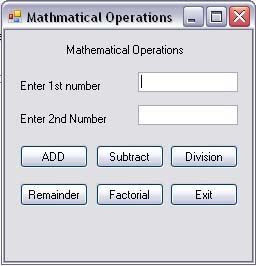
// Form1.designer.cs
namespace Mathematical_Operations
{
partial class Form1
{
/// < summary>
/// Required designer variable.
/// < /summary>
private System.ComponentModel.IContainer components = null;
/// < summary>
/// Clean up any resources being used.
/// < /summary>
/// < param name="disposing">true if managed resources should be disposed; otherwise, false.< /param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
/// < summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// < /summary>
private void InitializeComponent()
{
this.label1 = new System.Windows.Forms.Label();
this.label2 = new System.Windows.Forms.Label();
this.textBox1 = new System.Windows.Forms.TextBox();
this.textBox2 = new System.Windows.Forms.TextBox();
this.label3 = new System.Windows.Forms.Label();
this.button1 = new System.Windows.Forms.Button();
this.button2 = new System.Windows.Forms.Button();
this.button3 = new System.Windows.Forms.Button();
this.button4 = new System.Windows.Forms.Button();
this.button5 = new System.Windows.Forms.Button();
this.button6 = new System.Windows.Forms.Button();
this.label4 = new System.Windows.Forms.Label();
this.SuspendLayout();
//
// label1
//
this.label1.AutoSize = true;
this.label1.Location = new System.Drawing.Point(12, 49);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(87, 13);
this.label1.TabIndex = 0;
this.label1.Text = "Enter 1st number";
//
// label2
//
this.label2.AutoSize = true;
this.label2.Location = new System.Drawing.Point(12, 82);
this.label2.Name = "label2";
this.label2.Size = new System.Drawing.Size(93, 13);
this.label2.TabIndex = 1;
this.label2.Text = "Enter 2nd Number";
//
// textBox1
//
this.textBox1.Location = new System.Drawing.Point(133, 42);
this.textBox1.Name = "textBox1";
this.textBox1.Size = new System.Drawing.Size(100, 20);
this.textBox1.TabIndex = 2;
//
// textBox2
//
this.textBox2.Location = new System.Drawing.Point(133, 75);
this.textBox2.Name = "textBox2";
this.textBox2.Size = new System.Drawing.Size(100, 20);
this.textBox2.TabIndex = 3;
//
// label3
//
this.label3.AutoSize = true;
this.label3.Location = new System.Drawing.Point(58, 13);
this.label3.Name = "label3";
this.label3.Size = new System.Drawing.Size(124, 13);
this.label3.TabIndex = 4;
this.label3.Text = "Mathematical Operations";
//
// button1
//
this.button1.Location = new System.Drawing.Point(15, 115);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(68, 23);
this.button1.TabIndex = 5;
this.button1.Text = "ADD";
this.button1.UseVisualStyleBackColor = true;
this.button1.Click += new System.EventHandler(this.button1_Click);
//
// button2
//
this.button2.Location = new System.Drawing.Point(91, 115);
this.button2.Name = "button2";
this.button2.Size = new System.Drawing.Size(68, 23);
this.button2.TabIndex = 6;
this.button2.Text = "Subtract";
this.button2.UseVisualStyleBackColor = true;
this.button2.Click += new System.EventHandler(this.button2_Click);
//
// button3
//
this.button3.Location = new System.Drawing.Point(165, 115);
this.button3.Name = "button3";
this.button3.Size = new System.Drawing.Size(68, 23);
this.button3.TabIndex = 7;
this.button3.Text = "Division";
this.button3.UseVisualStyleBackColor = true;
this.button3.Click += new System.EventHandler(this.button3_Click);
//
// button4
//
this.button4.Location = new System.Drawing.Point(15, 153);
this.button4.Name = "button4";
this.button4.Size = new System.Drawing.Size(68, 23);
this.button4.TabIndex = 8;
this.button4.Text = "Remainder";
this.button4.UseVisualStyleBackColor = true;
this.button4.Click += new System.EventHandler(this.button4_Click);
//
// button5
//
this.button5.Location = new System.Drawing.Point(91, 153);
this.button5.Name = "button5";
this.button5.Size = new System.Drawing.Size(68, 23);
this.button5.TabIndex = 9;
this.button5.Text = "Factorial";
this.button5.UseVisualStyleBackColor = true;
this.button5.Click += new System.EventHandler(this.button5_Click);
//
// button6
//
this.button6.Location = new System.Drawing.Point(165, 153);
this.button6.Name = "button6";
this.button6.Size = new System.Drawing.Size(68, 23);
this.button6.TabIndex = 10;
this.button6.Text = "Exit";
this.button6.UseVisualStyleBackColor = true;
this.button6.Click += new System.EventHandler(this.button6_Click);
//
// label4
//
this.label4.AutoSize = true;
this.label4.Location = new System.Drawing.Point(94, 196);
this.label4.Name = "label4";
this.label4.Size = new System.Drawing.Size(0, 13);
this.label4.TabIndex = 11;
//
// Form1
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(247, 231);
this.Controls.Add(this.label4);
this.Controls.Add(this.button6);
this.Controls.Add(this.button5);
this.Controls.Add(this.button4);
this.Controls.Add(this.button3);
this.Controls.Add(this.button2);
this.Controls.Add(this.button1);
this.Controls.Add(this.label3);
this.Controls.Add(this.textBox2);
this.Controls.Add(this.textBox1);
this.Controls.Add(this.label2);
this.Controls.Add(this.label1);
this.Name = "Form1";
this.Text = "Mathmatical Operations";
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private System.Windows.Forms.Label label1;
private System.Windows.Forms.Label label2;
private System.Windows.Forms.TextBox textBox1;
private System.Windows.Forms.TextBox textBox2;
private System.Windows.Forms.Label label3;
private System.Windows.Forms.Button button1;
private System.Windows.Forms.Button button2;
private System.Windows.Forms.Button button3;
private System.Windows.Forms.Button button4;
private System.Windows.Forms.Button button5;
private System.Windows.Forms.Button button6;
private System.Windows.Forms.Label label4;
}
}
//Form1.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace Mathematical_Operations
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
double a, b;
a = Convert.ToDouble(textBox1.Text);
b = Convert.ToDouble(textBox2.Text);
label4.Text = Convert.ToString(a + b);
}
private void button2_Click(object sender, EventArgs e)
{
double a, b;
a = Convert.ToDouble(textBox1.Text);
b = Convert.ToDouble(textBox2.Text);
label4.Text = Convert.ToString(a - b);
}
private void button3_Click(object sender, EventArgs e)
{
double a, b;
a = Convert.ToDouble(textBox1.Text);
b = Convert.ToDouble(textBox2.Text);
label4.Text = Convert.ToString(a / b);
}
private void button4_Click(object sender, EventArgs e)
{
double a, b;
a = Convert.ToDouble(textBox1.Text);
b = Convert.ToDouble(textBox2.Text);
label4.Text = Convert.ToString((int)a % (int)b);
}
private void button5_Click(object sender, EventArgs e)
{
double a, b;
a = Convert.ToDouble(textBox1.Text);
b = Convert.ToDouble(textBox2.Text);
int fact=1;
for (int i = 1; i < = a; i++)
fact *= i;
a = fact;
fact=1;
for(int i=1;i< b;i++)
fact *= i;
label4.Text = "1st Number : "+a+"\n2nd Number :"+fact;
}
private void button6_Click(object sender, EventArgs e)
{
Application.Exit();
}
}
}