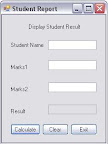
//Form1.designer.cs
namespace Student_Marks
{
partial class Form1
{
///
/// Required designer variable.
///
private System.ComponentModel.IContainer components = null;
///
/// Clean up any resources being used.
///
/// true if managed resources should be disposed; otherwise, false.
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
///
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
///
private void InitializeComponent()
{
this.label1 = new System.Windows.Forms.Label();
this.label2 = new System.Windows.Forms.Label();
this.label3 = new System.Windows.Forms.Label();
this.label4 = new System.Windows.Forms.Label();
this.button1 = new System.Windows.Forms.Button();
this.button2 = new System.Windows.Forms.Button();
this.textBox1 = new System.Windows.Forms.TextBox();
this.textBox2 = new System.Windows.Forms.TextBox();
this.textBox3 = new System.Windows.Forms.TextBox();
this.textBox4 = new System.Windows.Forms.TextBox();
this.button3 = new System.Windows.Forms.Button();
this.label5 = new System.Windows.Forms.Label();
this.SuspendLayout();
//
// label1
//
this.label1.AutoSize = true;
this.label1.Location = new System.Drawing.Point(15, 56);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(75, 13);
this.label1.TabIndex = 0;
this.label1.Text = "Student Name";
//
// label2
//
this.label2.AutoSize = true;
this.label2.Location = new System.Drawing.Point(15, 102);
this.label2.Name = "label2";
this.label2.Size = new System.Drawing.Size(42, 13);
this.label2.TabIndex = 1;
this.label2.Text = "Marks1";
//
// label3
//
this.label3.AutoSize = true;
this.label3.Location = new System.Drawing.Point(15, 148);
this.label3.Name = "label3";
this.label3.Size = new System.Drawing.Size(42, 13);
this.label3.TabIndex = 2;
this.label3.Text = "Marks2";
//
// label4
//
this.label4.AutoSize = true;
this.label4.Location = new System.Drawing.Point(15, 194);
this.label4.Name = "label4";
this.label4.Size = new System.Drawing.Size(37, 13);
this.label4.TabIndex = 3;
this.label4.Text = "Result";
//
// button1
//
this.button1.Location = new System.Drawing.Point(18, 227);
this.button1.Name = "button1";
this.button1.Size = new System.Drawing.Size(61, 23);
this.button1.TabIndex = 4;
this.button1.Text = "Calculate";
this.button1.UseVisualStyleBackColor = true;
this.button1.Click += new System.EventHandler(this.button1_Click);
//
// button2
//
this.button2.Location = new System.Drawing.Point(84, 227);
this.button2.Name = "button2";
this.button2.Size = new System.Drawing.Size(54, 23);
this.button2.TabIndex = 5;
this.button2.Text = "Clear";
this.button2.UseVisualStyleBackColor = true;
this.button2.Click += new System.EventHandler(this.button2_Click);
//
// textBox1
//
this.textBox1.Location = new System.Drawing.Point(96, 53);
this.textBox1.Name = "textBox1";
this.textBox1.Size = new System.Drawing.Size(100, 20);
this.textBox1.TabIndex = 6;
//
// textBox2
//
this.textBox2.Location = new System.Drawing.Point(96, 95);
this.textBox2.Name = "textBox2";
this.textBox2.Size = new System.Drawing.Size(100, 20);
this.textBox2.TabIndex = 7;
//
// textBox3
//
this.textBox3.Location = new System.Drawing.Point(96, 141);
this.textBox3.Name = "textBox3";
this.textBox3.Size = new System.Drawing.Size(100, 20);
this.textBox3.TabIndex = 8;
//
// textBox4
//
this.textBox4.Enabled = false;
this.textBox4.Location = new System.Drawing.Point(96, 187);
this.textBox4.Name = "textBox4";
this.textBox4.Size = new System.Drawing.Size(100, 20);
this.textBox4.TabIndex = 9;
//
// button3
//
this.button3.Location = new System.Drawing.Point(143, 227);
this.button3.Name = "button3";
this.button3.Size = new System.Drawing.Size(54, 23);
this.button3.TabIndex = 10;
this.button3.Text = "Exit";
this.button3.UseVisualStyleBackColor = true;
this.button3.Click += new System.EventHandler(this.button3_Click);
//
// label5
//
this.label5.AutoSize = true;
this.label5.Location = new System.Drawing.Point(52, 18);
this.label5.Name = "label5";
this.label5.Size = new System.Drawing.Size(114, 13);
this.label5.TabIndex = 11;
this.label5.Text = "Display Student Result";
//
// Form1
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(215, 266);
this.Controls.Add(this.label5);
this.Controls.Add(this.button3);
this.Controls.Add(this.textBox4);
this.Controls.Add(this.textBox3);
this.Controls.Add(this.textBox2);
this.Controls.Add(this.textBox1);
this.Controls.Add(this.button2);
this.Controls.Add(this.button1);
this.Controls.Add(this.label4);
this.Controls.Add(this.label3);
this.Controls.Add(this.label2);
this.Controls.Add(this.label1);
this.Name = "Form1";
this.Text = "Student Report";
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private System.Windows.Forms.Label label1;
private System.Windows.Forms.Label label2;
private System.Windows.Forms.Label label3;
private System.Windows.Forms.Label label4;
private System.Windows.Forms.Button button1;
private System.Windows.Forms.Button button2;
private System.Windows.Forms.TextBox textBox1;
private System.Windows.Forms.TextBox textBox2;
private System.Windows.Forms.TextBox textBox3;
private System.Windows.Forms.TextBox textBox4;
private System.Windows.Forms.Button button3;
private System.Windows.Forms.Label label5;
}
}
// Form1.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace Student_Marks
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button3_Click(object sender, EventArgs e)
{
Application.Exit();
/*Form2 form = new Form2();
form.Show();*/
}
private void button2_Click(object sender, EventArgs e)
{
textBox1.Text = "";
textBox2.Text = "";
textBox3.Text = "";
textBox4.Text = "";
}
private void button1_Click(object sender, EventArgs e)
{
int marks1 = Convert.ToInt32(textBox2.Text);
int marks2 = Convert.ToInt32(textBox3.Text);
textBox4.Text = Convert.ToString(marks1 + marks2);
}
private void button4_Click(object sender, EventArgs e)
{
}
}
}
No comments:
Post a Comment